2021-04-08 12:41:57 +02:00
|
|
|
import fastGlob from 'fast-glob';
|
|
|
|
import wrapAnsi from 'wrap-ansi';
|
|
|
|
import AddAssetPlugin from 'add-asset-webpack-plugin';
|
|
|
|
import LicenseCheckerWebpackPlugin from 'license-checker-webpack-plugin';
|
|
|
|
import MiniCssExtractPlugin from 'mini-css-extract-plugin';
|
|
|
|
import MonacoWebpackPlugin from 'monaco-editor-webpack-plugin';
|
2022-10-01 16:26:38 +02:00
|
|
|
import {VueLoaderPlugin} from 'vue-loader';
|
2021-04-15 22:07:31 +02:00
|
|
|
import EsBuildLoader from 'esbuild-loader';
|
2022-12-20 23:15:47 +01:00
|
|
|
import {parse, dirname} from 'node:path';
|
2021-04-08 12:41:57 +02:00
|
|
|
import webpack from 'webpack';
|
2022-12-20 23:15:47 +01:00
|
|
|
import {fileURLToPath} from 'node:url';
|
|
|
|
import {readFileSync} from 'node:fs';
|
2023-06-06 06:57:08 +02:00
|
|
|
import {env} from 'node:process';
|
Add tailwindcss (#29357)
This will get tailwindcss working on a basic level. It provides only the
utility classes, e.g. no tailwind base which we don't need because we
have our own CSS reset. Without the base, we also do not have their CSS
variables so a small amount of features do not work and I removed the
generated classes for them.
***Note for future developers: This currently uses a `tw-` prefix, so we
use it like `tw-p-3`.***
<details>
<summary>Currently added CSS, all false-positives</summary>
```
.\!visible{
visibility: visible !important
}
.visible{
visibility: visible
}
.invisible{
visibility: hidden
}
.collapse{
visibility: collapse
}
.static{
position: static
}
.\!fixed{
position: fixed !important
}
.absolute{
position: absolute
}
.relative{
position: relative
}
.sticky{
position: sticky
}
.left-10{
left: 2.5rem
}
.isolate{
isolation: isolate
}
.float-right{
float: right
}
.float-left{
float: left
}
.mr-2{
margin-right: 0.5rem
}
.mr-3{
margin-right: 0.75rem
}
.\!block{
display: block !important
}
.block{
display: block
}
.inline-block{
display: inline-block
}
.inline{
display: inline
}
.flex{
display: flex
}
.inline-flex{
display: inline-flex
}
.\!table{
display: table !important
}
.inline-table{
display: inline-table
}
.table-caption{
display: table-caption
}
.table-cell{
display: table-cell
}
.table-column{
display: table-column
}
.table-column-group{
display: table-column-group
}
.table-footer-group{
display: table-footer-group
}
.table-header-group{
display: table-header-group
}
.table-row-group{
display: table-row-group
}
.table-row{
display: table-row
}
.flow-root{
display: flow-root
}
.inline-grid{
display: inline-grid
}
.contents{
display: contents
}
.list-item{
display: list-item
}
.\!hidden{
display: none !important
}
.hidden{
display: none
}
.flex-shrink{
flex-shrink: 1
}
.shrink{
flex-shrink: 1
}
.flex-grow{
flex-grow: 1
}
.grow{
flex-grow: 1
}
.border-collapse{
border-collapse: collapse
}
.select-all{
user-select: all
}
.resize{
resize: both
}
.flex-wrap{
flex-wrap: wrap
}
.overflow-visible{
overflow: visible
}
.rounded{
border-radius: 0.25rem
}
.border{
border-width: 1px
}
.text-justify{
text-align: justify
}
.uppercase{
text-transform: uppercase
}
.lowercase{
text-transform: lowercase
}
.capitalize{
text-transform: capitalize
}
.italic{
font-style: italic
}
.text-red{
color: var(--color-red)
}
.text-shadow{
color: var(--color-shadow)
}
.underline{
text-decoration-line: underline
}
.overline{
text-decoration-line: overline
}
.line-through{
text-decoration-line: line-through
}
.outline{
outline-style: solid
}
.ease-in{
transition-timing-function: cubic-bezier(0.4, 0, 1, 1)
}
.ease-in-out{
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1)
}
.ease-out{
transition-timing-function: cubic-bezier(0, 0, 0.2, 1)
}
```
</details>
---------
Co-authored-by: Giteabot <teabot@gitea.io>
2024-02-25 17:46:46 +01:00
|
|
|
import tailwindcss from 'tailwindcss';
|
|
|
|
import tailwindConfig from './tailwind.config.js';
|
2024-03-14 19:20:54 +01:00
|
|
|
import tailwindcssNesting from 'tailwindcss/nesting/index.js';
|
|
|
|
import postcssNesting from 'postcss-nesting';
|
2021-04-08 12:41:57 +02:00
|
|
|
|
2023-03-17 06:45:45 +01:00
|
|
|
const {EsbuildPlugin} = EsBuildLoader;
|
|
|
|
const {SourceMapDevToolPlugin, DefinePlugin} = webpack;
|
2022-09-07 23:35:54 +02:00
|
|
|
const formatLicenseText = (licenseText) => wrapAnsi(licenseText || '', 80).trim();
|
|
|
|
|
2022-06-06 05:27:25 +02:00
|
|
|
const glob = (pattern) => fastGlob.sync(pattern, {
|
|
|
|
cwd: dirname(fileURLToPath(new URL(import.meta.url))),
|
|
|
|
absolute: true,
|
|
|
|
});
|
2020-02-11 18:02:41 +01:00
|
|
|
|
2020-01-28 08:30:39 +01:00
|
|
|
const themes = {};
|
2023-03-15 03:20:19 +01:00
|
|
|
for (const path of glob('web_src/css/themes/*.css')) {
|
2020-01-28 08:30:39 +01:00
|
|
|
themes[parse(path).name] = [path];
|
|
|
|
}
|
2019-11-13 15:52:13 +01:00
|
|
|
|
2023-06-06 06:57:08 +02:00
|
|
|
const isProduction = env.NODE_ENV !== 'development';
|
|
|
|
|
2023-09-27 04:05:49 +02:00
|
|
|
// ENABLE_SOURCEMAP accepts the following values:
|
|
|
|
// true - all enabled, the default in development
|
|
|
|
// reduced - minimal sourcemaps, the default in production
|
|
|
|
// false - all disabled
|
|
|
|
let sourceMaps;
|
2023-06-06 06:57:08 +02:00
|
|
|
if ('ENABLE_SOURCEMAP' in env) {
|
2023-09-27 04:05:49 +02:00
|
|
|
sourceMaps = ['true', 'false'].includes(env.ENABLE_SOURCEMAP) ? env.ENABLE_SOURCEMAP : 'reduced';
|
2023-06-06 06:57:08 +02:00
|
|
|
} else {
|
2023-09-27 04:05:49 +02:00
|
|
|
sourceMaps = isProduction ? 'reduced' : 'true';
|
2023-06-06 06:57:08 +02:00
|
|
|
}
|
2020-02-23 09:47:42 +01:00
|
|
|
|
2024-03-15 03:05:31 +01:00
|
|
|
// define which web components we use for Vue to not interpret them as Vue components
|
|
|
|
const webComponents = new Set([
|
|
|
|
// our own, in web_src/js/webcomponents
|
|
|
|
'overflow-menu',
|
|
|
|
'origin-url',
|
|
|
|
'absolute-date',
|
|
|
|
// from dependencies
|
|
|
|
'markdown-toolbar',
|
|
|
|
'relative-time',
|
|
|
|
'text-expander',
|
|
|
|
]);
|
|
|
|
|
2020-07-27 23:01:25 +02:00
|
|
|
const filterCssImport = (url, ...args) => {
|
|
|
|
const cssFile = args[1] || args[0]; // resourcePath is 2nd argument for url and 3rd for import
|
2020-06-28 04:59:56 +02:00
|
|
|
const importedFile = url.replace(/[?#].+/, '').toLowerCase();
|
2020-07-06 10:56:54 +02:00
|
|
|
|
2020-06-28 04:59:56 +02:00
|
|
|
if (cssFile.includes('fomantic')) {
|
|
|
|
if (/brand-icons/.test(importedFile)) return false;
|
2023-09-01 16:07:37 +02:00
|
|
|
if (/(eot|ttf|otf|woff|svg)$/i.test(importedFile)) return false;
|
2020-07-06 10:56:54 +02:00
|
|
|
}
|
|
|
|
|
2023-09-01 16:07:37 +02:00
|
|
|
if (cssFile.includes('katex') && /(ttf|woff)$/i.test(importedFile)) {
|
2022-09-13 18:33:37 +02:00
|
|
|
return false;
|
|
|
|
}
|
|
|
|
|
2020-07-06 10:56:54 +02:00
|
|
|
return true;
|
2020-06-28 04:59:56 +02:00
|
|
|
};
|
|
|
|
|
2022-11-18 18:01:06 +01:00
|
|
|
/** @type {import("webpack").Configuration} */
|
2021-04-08 12:41:57 +02:00
|
|
|
export default {
|
2020-02-23 09:47:42 +01:00
|
|
|
mode: isProduction ? 'production' : 'development',
|
2019-11-13 15:52:13 +01:00
|
|
|
entry: {
|
2020-01-28 08:30:39 +01:00
|
|
|
index: [
|
2022-06-06 05:27:25 +02:00
|
|
|
fileURLToPath(new URL('web_src/js/jquery.js', import.meta.url)),
|
|
|
|
fileURLToPath(new URL('web_src/fomantic/build/semantic.js', import.meta.url)),
|
|
|
|
fileURLToPath(new URL('web_src/js/index.js', import.meta.url)),
|
|
|
|
fileURLToPath(new URL('node_modules/easymde/dist/easymde.min.css', import.meta.url)),
|
|
|
|
fileURLToPath(new URL('web_src/fomantic/build/semantic.css', import.meta.url)),
|
2023-03-15 03:20:19 +01:00
|
|
|
fileURLToPath(new URL('web_src/css/index.css', import.meta.url)),
|
2020-01-28 08:30:39 +01:00
|
|
|
],
|
Introduce customized HTML elements, fix incorrect AppUrl usages in templates (#22861)
This PR follows:
* #21986
* #22831
This PR also introduce customized HTML elements, which would also help
problems like:
* #17760
* #21429
* #21440
With customized HTML elements, there won't be any load-search-replace
operations, and it can avoid page flicking (which @silverwind cares a
lot).
Browser support:
https://developer.mozilla.org/en-US/docs/Web/API/Window/customElements
# FAQ
## Why the component has the prefix?
As usual, I would strongly suggest to add prefixes for our own/private
names. The dedicated prefix will avoid conflicts in the future, and it
makes it easier to introduce various 3rd components, like GitHub's
`relative-time` component. If there is no prefix, it's impossible to
introduce another public component with the same name in the future.
## Why the `custcomp.js` is loaded before HTML body? The `index.js` is
after HTML body.
Customized components must be registered before the content loading.
Otherwise there would be still some flicking.
`custcomp.js` should have its own dependencies and should be very light,
so it won't affect the page loading time too much.
## Why use `data-url` attribute but not use the `textContent`?
According to the standard, the `connectedCallback` occurs on the
tag-opening moment. The element's children are not ready yet.
## Why not use `{{.GuessCurrentOrigin $.ctx ...}}` to let backend decide
the absolute URL?
It's difficult for backend to guess the correct protocol(scheme)
correctly with zero configuration. Generating the absolute URL from
frontend can guarantee that the URL is 100% correct -- since the user is
visiting it.
# Screenshot
<details>
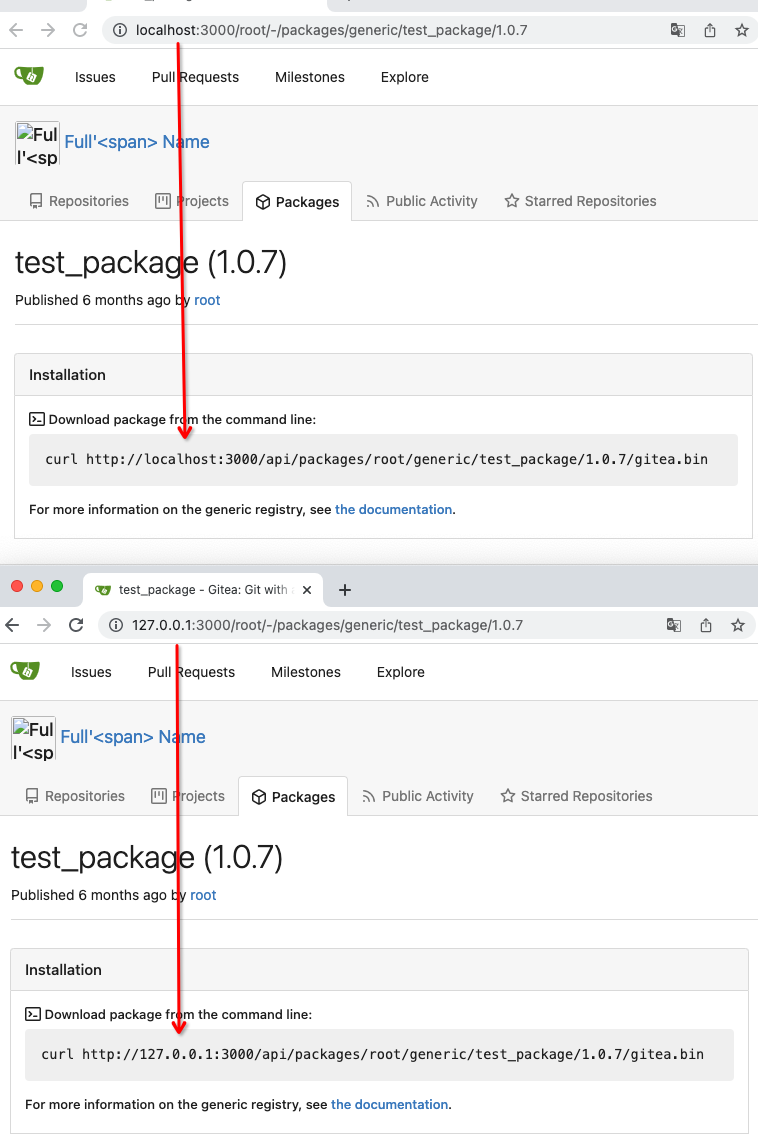
</details>
2023-02-17 15:02:20 +01:00
|
|
|
webcomponents: [
|
2024-03-15 03:05:31 +01:00
|
|
|
fileURLToPath(new URL('web_src/js/webcomponents/index.js', import.meta.url)),
|
Introduce customized HTML elements, fix incorrect AppUrl usages in templates (#22861)
This PR follows:
* #21986
* #22831
This PR also introduce customized HTML elements, which would also help
problems like:
* #17760
* #21429
* #21440
With customized HTML elements, there won't be any load-search-replace
operations, and it can avoid page flicking (which @silverwind cares a
lot).
Browser support:
https://developer.mozilla.org/en-US/docs/Web/API/Window/customElements
# FAQ
## Why the component has the prefix?
As usual, I would strongly suggest to add prefixes for our own/private
names. The dedicated prefix will avoid conflicts in the future, and it
makes it easier to introduce various 3rd components, like GitHub's
`relative-time` component. If there is no prefix, it's impossible to
introduce another public component with the same name in the future.
## Why the `custcomp.js` is loaded before HTML body? The `index.js` is
after HTML body.
Customized components must be registered before the content loading.
Otherwise there would be still some flicking.
`custcomp.js` should have its own dependencies and should be very light,
so it won't affect the page loading time too much.
## Why use `data-url` attribute but not use the `textContent`?
According to the standard, the `connectedCallback` occurs on the
tag-opening moment. The element's children are not ready yet.
## Why not use `{{.GuessCurrentOrigin $.ctx ...}}` to let backend decide
the absolute URL?
It's difficult for backend to guess the correct protocol(scheme)
correctly with zero configuration. Generating the absolute URL from
frontend can guarantee that the URL is 100% correct -- since the user is
visiting it.
# Screenshot
<details>
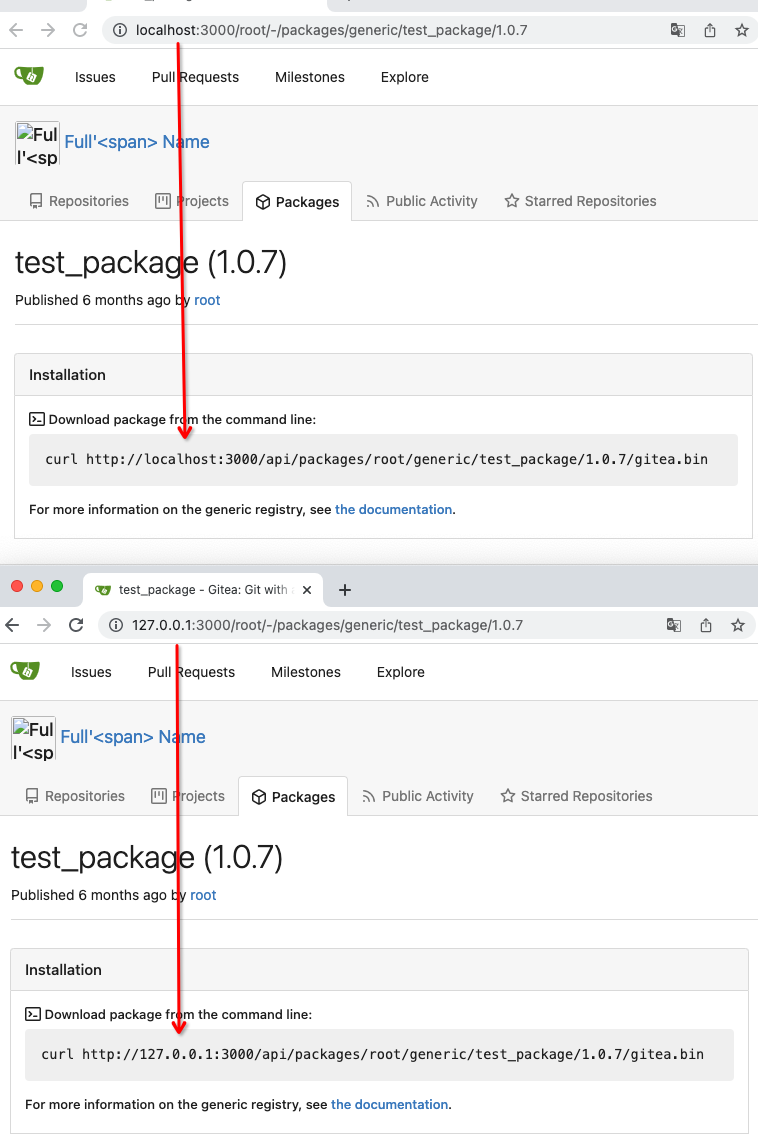
</details>
2023-02-17 15:02:20 +01:00
|
|
|
],
|
2020-01-28 08:30:39 +01:00
|
|
|
swagger: [
|
2022-06-06 05:27:25 +02:00
|
|
|
fileURLToPath(new URL('web_src/js/standalone/swagger.js', import.meta.url)),
|
2023-03-15 03:20:19 +01:00
|
|
|
fileURLToPath(new URL('web_src/css/standalone/swagger.css', import.meta.url)),
|
2020-01-28 08:30:39 +01:00
|
|
|
],
|
2020-07-03 11:55:36 +02:00
|
|
|
'eventsource.sharedworker': [
|
2022-06-06 05:27:25 +02:00
|
|
|
fileURLToPath(new URL('web_src/js/features/eventsource.sharedworker.js', import.meta.url)),
|
2020-07-03 11:55:36 +02:00
|
|
|
],
|
2023-06-27 04:45:24 +02:00
|
|
|
...(!isProduction && {
|
|
|
|
devtest: [
|
|
|
|
fileURLToPath(new URL('web_src/js/standalone/devtest.js', import.meta.url)),
|
|
|
|
fileURLToPath(new URL('web_src/css/standalone/devtest.css', import.meta.url)),
|
|
|
|
],
|
|
|
|
}),
|
2020-01-28 08:30:39 +01:00
|
|
|
...themes,
|
2019-11-13 15:52:13 +01:00
|
|
|
},
|
2020-01-14 19:02:08 +01:00
|
|
|
devtool: false,
|
2019-11-13 15:52:13 +01:00
|
|
|
output: {
|
2023-07-18 18:06:43 +02:00
|
|
|
path: fileURLToPath(new URL('public/assets', import.meta.url)),
|
2023-05-31 04:07:04 +02:00
|
|
|
filename: () => 'js/[name].js',
|
2020-12-27 15:24:27 +01:00
|
|
|
chunkFilename: ({chunk}) => {
|
|
|
|
const language = (/monaco.*languages?_.+?_(.+?)_/.exec(chunk.id) || [])[1];
|
2022-08-23 14:58:04 +02:00
|
|
|
return `js/${language ? `monaco-language-${language.toLowerCase()}` : `[name]`}.[contenthash:8].js`;
|
2020-12-27 15:24:27 +01:00
|
|
|
},
|
2019-11-13 15:52:13 +01:00
|
|
|
},
|
|
|
|
optimization: {
|
2020-02-23 09:47:42 +01:00
|
|
|
minimize: isProduction,
|
2020-01-25 09:41:34 +01:00
|
|
|
minimizer: [
|
2023-03-17 06:45:45 +01:00
|
|
|
new EsbuildPlugin({
|
2024-02-02 10:36:32 +01:00
|
|
|
target: 'es2020',
|
2021-05-07 21:12:37 +02:00
|
|
|
minify: true,
|
2024-02-07 03:17:59 +01:00
|
|
|
css: true,
|
2021-05-19 23:46:30 +02:00
|
|
|
legalComments: 'none',
|
2020-01-25 09:41:34 +01:00
|
|
|
}),
|
|
|
|
],
|
2020-01-28 22:57:20 +01:00
|
|
|
splitChunks: {
|
|
|
|
chunks: 'async',
|
|
|
|
name: (_, chunks) => chunks.map((item) => item.name).join('-'),
|
2020-06-28 04:59:56 +02:00
|
|
|
},
|
2020-12-27 15:24:27 +01:00
|
|
|
moduleIds: 'named',
|
|
|
|
chunkIds: 'named',
|
2019-11-13 15:52:13 +01:00
|
|
|
},
|
2019-11-14 22:39:51 +01:00
|
|
|
module: {
|
|
|
|
rules: [
|
2020-01-20 11:07:30 +01:00
|
|
|
{
|
2023-09-01 16:07:37 +02:00
|
|
|
test: /\.vue$/i,
|
2020-01-20 11:07:30 +01:00
|
|
|
exclude: /node_modules/,
|
2020-01-25 09:41:34 +01:00
|
|
|
loader: 'vue-loader',
|
2024-03-15 03:05:31 +01:00
|
|
|
options: {
|
|
|
|
compilerOptions: {
|
|
|
|
isCustomElement: (tag) => webComponents.has(tag),
|
|
|
|
},
|
|
|
|
},
|
2020-01-20 11:07:30 +01:00
|
|
|
},
|
2019-11-14 22:39:51 +01:00
|
|
|
{
|
2023-09-01 16:07:37 +02:00
|
|
|
test: /\.js$/i,
|
2019-11-14 22:39:51 +01:00
|
|
|
exclude: /node_modules/,
|
2020-01-22 07:35:29 +01:00
|
|
|
use: [
|
|
|
|
{
|
2021-04-02 02:11:04 +02:00
|
|
|
loader: 'esbuild-loader',
|
2020-01-22 07:35:29 +01:00
|
|
|
options: {
|
2023-03-17 06:45:45 +01:00
|
|
|
loader: 'js',
|
2024-02-02 15:01:32 +01:00
|
|
|
target: 'es2020',
|
2020-01-25 09:41:34 +01:00
|
|
|
},
|
2020-01-22 07:35:29 +01:00
|
|
|
},
|
|
|
|
],
|
2019-11-17 22:39:06 +01:00
|
|
|
},
|
|
|
|
{
|
2023-03-15 03:20:19 +01:00
|
|
|
test: /\.css$/i,
|
2020-06-28 04:59:56 +02:00
|
|
|
use: [
|
|
|
|
{
|
|
|
|
loader: MiniCssExtractPlugin.loader,
|
|
|
|
},
|
|
|
|
{
|
|
|
|
loader: 'css-loader',
|
|
|
|
options: {
|
2023-09-27 04:05:49 +02:00
|
|
|
sourceMap: sourceMaps === 'true',
|
2021-08-17 07:32:48 +02:00
|
|
|
url: {filter: filterCssImport},
|
|
|
|
import: {filter: filterCssImport},
|
2024-03-14 19:20:54 +01:00
|
|
|
importLoaders: 1,
|
2020-06-28 04:59:56 +02:00
|
|
|
},
|
|
|
|
},
|
Add tailwindcss (#29357)
This will get tailwindcss working on a basic level. It provides only the
utility classes, e.g. no tailwind base which we don't need because we
have our own CSS reset. Without the base, we also do not have their CSS
variables so a small amount of features do not work and I removed the
generated classes for them.
***Note for future developers: This currently uses a `tw-` prefix, so we
use it like `tw-p-3`.***
<details>
<summary>Currently added CSS, all false-positives</summary>
```
.\!visible{
visibility: visible !important
}
.visible{
visibility: visible
}
.invisible{
visibility: hidden
}
.collapse{
visibility: collapse
}
.static{
position: static
}
.\!fixed{
position: fixed !important
}
.absolute{
position: absolute
}
.relative{
position: relative
}
.sticky{
position: sticky
}
.left-10{
left: 2.5rem
}
.isolate{
isolation: isolate
}
.float-right{
float: right
}
.float-left{
float: left
}
.mr-2{
margin-right: 0.5rem
}
.mr-3{
margin-right: 0.75rem
}
.\!block{
display: block !important
}
.block{
display: block
}
.inline-block{
display: inline-block
}
.inline{
display: inline
}
.flex{
display: flex
}
.inline-flex{
display: inline-flex
}
.\!table{
display: table !important
}
.inline-table{
display: inline-table
}
.table-caption{
display: table-caption
}
.table-cell{
display: table-cell
}
.table-column{
display: table-column
}
.table-column-group{
display: table-column-group
}
.table-footer-group{
display: table-footer-group
}
.table-header-group{
display: table-header-group
}
.table-row-group{
display: table-row-group
}
.table-row{
display: table-row
}
.flow-root{
display: flow-root
}
.inline-grid{
display: inline-grid
}
.contents{
display: contents
}
.list-item{
display: list-item
}
.\!hidden{
display: none !important
}
.hidden{
display: none
}
.flex-shrink{
flex-shrink: 1
}
.shrink{
flex-shrink: 1
}
.flex-grow{
flex-grow: 1
}
.grow{
flex-grow: 1
}
.border-collapse{
border-collapse: collapse
}
.select-all{
user-select: all
}
.resize{
resize: both
}
.flex-wrap{
flex-wrap: wrap
}
.overflow-visible{
overflow: visible
}
.rounded{
border-radius: 0.25rem
}
.border{
border-width: 1px
}
.text-justify{
text-align: justify
}
.uppercase{
text-transform: uppercase
}
.lowercase{
text-transform: lowercase
}
.capitalize{
text-transform: capitalize
}
.italic{
font-style: italic
}
.text-red{
color: var(--color-red)
}
.text-shadow{
color: var(--color-shadow)
}
.underline{
text-decoration-line: underline
}
.overline{
text-decoration-line: overline
}
.line-through{
text-decoration-line: line-through
}
.outline{
outline-style: solid
}
.ease-in{
transition-timing-function: cubic-bezier(0.4, 0, 1, 1)
}
.ease-in-out{
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1)
}
.ease-out{
transition-timing-function: cubic-bezier(0, 0, 0.2, 1)
}
```
</details>
---------
Co-authored-by: Giteabot <teabot@gitea.io>
2024-02-25 17:46:46 +01:00
|
|
|
{
|
|
|
|
loader: 'postcss-loader',
|
|
|
|
options: {
|
|
|
|
postcssOptions: {
|
2024-03-14 19:20:54 +01:00
|
|
|
plugins: [
|
|
|
|
tailwindcssNesting(postcssNesting({edition: '2024-02'})),
|
|
|
|
tailwindcss(tailwindConfig),
|
|
|
|
],
|
Add tailwindcss (#29357)
This will get tailwindcss working on a basic level. It provides only the
utility classes, e.g. no tailwind base which we don't need because we
have our own CSS reset. Without the base, we also do not have their CSS
variables so a small amount of features do not work and I removed the
generated classes for them.
***Note for future developers: This currently uses a `tw-` prefix, so we
use it like `tw-p-3`.***
<details>
<summary>Currently added CSS, all false-positives</summary>
```
.\!visible{
visibility: visible !important
}
.visible{
visibility: visible
}
.invisible{
visibility: hidden
}
.collapse{
visibility: collapse
}
.static{
position: static
}
.\!fixed{
position: fixed !important
}
.absolute{
position: absolute
}
.relative{
position: relative
}
.sticky{
position: sticky
}
.left-10{
left: 2.5rem
}
.isolate{
isolation: isolate
}
.float-right{
float: right
}
.float-left{
float: left
}
.mr-2{
margin-right: 0.5rem
}
.mr-3{
margin-right: 0.75rem
}
.\!block{
display: block !important
}
.block{
display: block
}
.inline-block{
display: inline-block
}
.inline{
display: inline
}
.flex{
display: flex
}
.inline-flex{
display: inline-flex
}
.\!table{
display: table !important
}
.inline-table{
display: inline-table
}
.table-caption{
display: table-caption
}
.table-cell{
display: table-cell
}
.table-column{
display: table-column
}
.table-column-group{
display: table-column-group
}
.table-footer-group{
display: table-footer-group
}
.table-header-group{
display: table-header-group
}
.table-row-group{
display: table-row-group
}
.table-row{
display: table-row
}
.flow-root{
display: flow-root
}
.inline-grid{
display: inline-grid
}
.contents{
display: contents
}
.list-item{
display: list-item
}
.\!hidden{
display: none !important
}
.hidden{
display: none
}
.flex-shrink{
flex-shrink: 1
}
.shrink{
flex-shrink: 1
}
.flex-grow{
flex-grow: 1
}
.grow{
flex-grow: 1
}
.border-collapse{
border-collapse: collapse
}
.select-all{
user-select: all
}
.resize{
resize: both
}
.flex-wrap{
flex-wrap: wrap
}
.overflow-visible{
overflow: visible
}
.rounded{
border-radius: 0.25rem
}
.border{
border-width: 1px
}
.text-justify{
text-align: justify
}
.uppercase{
text-transform: uppercase
}
.lowercase{
text-transform: lowercase
}
.capitalize{
text-transform: capitalize
}
.italic{
font-style: italic
}
.text-red{
color: var(--color-red)
}
.text-shadow{
color: var(--color-shadow)
}
.underline{
text-decoration-line: underline
}
.overline{
text-decoration-line: overline
}
.line-through{
text-decoration-line: line-through
}
.outline{
outline-style: solid
}
.ease-in{
transition-timing-function: cubic-bezier(0.4, 0, 1, 1)
}
.ease-in-out{
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1)
}
.ease-out{
transition-timing-function: cubic-bezier(0, 0, 0.2, 1)
}
```
</details>
---------
Co-authored-by: Giteabot <teabot@gitea.io>
2024-02-25 17:46:46 +01:00
|
|
|
},
|
|
|
|
},
|
2024-03-22 15:06:53 +01:00
|
|
|
},
|
2020-06-28 04:59:56 +02:00
|
|
|
],
|
|
|
|
},
|
2020-02-11 18:02:41 +01:00
|
|
|
{
|
2023-09-01 16:07:37 +02:00
|
|
|
test: /\.svg$/i,
|
2023-07-18 18:06:43 +02:00
|
|
|
include: fileURLToPath(new URL('public/assets/img/svg', import.meta.url)),
|
2021-03-22 23:10:09 +01:00
|
|
|
type: 'asset/source',
|
2020-02-11 18:02:41 +01:00
|
|
|
},
|
2020-05-14 18:06:01 +02:00
|
|
|
{
|
2023-09-01 16:07:37 +02:00
|
|
|
test: /\.(ttf|woff2?)$/i,
|
2021-03-30 13:17:24 +02:00
|
|
|
type: 'asset/resource',
|
|
|
|
generator: {
|
2022-08-23 14:58:04 +02:00
|
|
|
filename: 'fonts/[name].[contenthash:8][ext]',
|
2024-03-22 15:06:53 +01:00
|
|
|
},
|
2020-07-29 20:44:23 +02:00
|
|
|
},
|
|
|
|
{
|
|
|
|
test: /\.png$/i,
|
2021-03-30 13:17:24 +02:00
|
|
|
type: 'asset/resource',
|
|
|
|
generator: {
|
2022-08-23 14:58:04 +02:00
|
|
|
filename: 'img/webpack/[name].[contenthash:8][ext]',
|
2024-03-22 15:06:53 +01:00
|
|
|
},
|
2020-05-14 18:06:01 +02:00
|
|
|
},
|
2020-01-25 09:41:34 +01:00
|
|
|
],
|
2020-01-14 19:02:08 +01:00
|
|
|
},
|
|
|
|
plugins: [
|
2024-02-16 03:52:25 +01:00
|
|
|
new webpack.ProvidePlugin({ // for htmx extensions
|
|
|
|
htmx: 'htmx.org',
|
|
|
|
}),
|
2023-03-17 06:45:45 +01:00
|
|
|
new DefinePlugin({
|
2023-03-14 10:51:20 +01:00
|
|
|
__VUE_OPTIONS_API__: true, // at the moment, many Vue components still use the Vue Options API
|
|
|
|
__VUE_PROD_DEVTOOLS__: false, // do not enable devtools support in production
|
2024-02-16 03:27:45 +01:00
|
|
|
__VUE_PROD_HYDRATION_MISMATCH_DETAILS__: false, // https://github.com/vuejs/vue-cli/pull/7443
|
2023-03-14 10:51:20 +01:00
|
|
|
}),
|
2020-01-20 11:07:30 +01:00
|
|
|
new VueLoaderPlugin(),
|
2020-01-25 09:41:34 +01:00
|
|
|
new MiniCssExtractPlugin({
|
|
|
|
filename: 'css/[name].css',
|
2022-08-23 14:58:04 +02:00
|
|
|
chunkFilename: 'css/[name].[contenthash:8].css',
|
2020-01-25 09:41:34 +01:00
|
|
|
}),
|
2023-09-27 04:05:49 +02:00
|
|
|
sourceMaps !== 'false' && new SourceMapDevToolPlugin({
|
2022-08-23 14:58:04 +02:00
|
|
|
filename: '[file].[contenthash:8].map',
|
2023-09-27 04:05:49 +02:00
|
|
|
...(sourceMaps === 'reduced' && {include: /^js\/index\.js$/}),
|
|
|
|
}),
|
2020-05-14 18:06:01 +02:00
|
|
|
new MonacoWebpackPlugin({
|
2022-08-23 14:58:04 +02:00
|
|
|
filename: 'js/monaco-[name].[contenthash:8].worker.js',
|
2020-05-14 18:06:01 +02:00
|
|
|
}),
|
2020-12-27 15:24:27 +01:00
|
|
|
isProduction ? new LicenseCheckerWebpackPlugin({
|
2023-09-01 16:42:33 +02:00
|
|
|
outputFilename: 'licenses.txt',
|
2020-12-27 15:24:27 +01:00
|
|
|
outputWriter: ({dependencies}) => {
|
2020-06-12 13:26:37 +02:00
|
|
|
const line = '-'.repeat(80);
|
2022-09-07 23:35:54 +02:00
|
|
|
const goJson = readFileSync('assets/go-licenses.json', 'utf8');
|
|
|
|
const goModules = JSON.parse(goJson).map(({name, licenseText}) => {
|
|
|
|
return {name, body: formatLicenseText(licenseText)};
|
|
|
|
});
|
2022-09-04 00:20:46 +02:00
|
|
|
const jsModules = dependencies.map(({name, version, licenseName, licenseText}) => {
|
2022-09-07 23:35:54 +02:00
|
|
|
return {name, version, licenseName, body: formatLicenseText(licenseText)};
|
2022-09-04 00:20:46 +02:00
|
|
|
});
|
|
|
|
|
|
|
|
const modules = [...goModules, ...jsModules].sort((a, b) => a.name.localeCompare(b.name));
|
|
|
|
return modules.map(({name, version, licenseName, body}) => {
|
|
|
|
const title = licenseName ? `${name}@${version} - ${licenseName}` : name;
|
|
|
|
return `${line}\n${title}\n${line}\n${body}`;
|
2020-06-12 13:26:37 +02:00
|
|
|
}).join('\n');
|
|
|
|
},
|
2020-12-27 15:24:27 +01:00
|
|
|
override: {
|
2022-11-22 01:58:55 +01:00
|
|
|
'khroma@*': {licenseName: 'MIT'}, // https://github.com/fabiospampinato/khroma/pull/33
|
2024-03-15 19:24:27 +01:00
|
|
|
'idiomorph@0.3.0': {licenseName: 'BSD-2-Clause'}, // https://github.com/bigskysoftware/idiomorph/pull/37
|
2020-06-12 13:26:37 +02:00
|
|
|
},
|
2022-11-18 18:54:32 +01:00
|
|
|
emitError: true,
|
2024-03-15 19:24:27 +01:00
|
|
|
allow: '(Apache-2.0 OR 0BSD OR BSD-2-Clause OR BSD-3-Clause OR MIT OR ISC OR CPAL-1.0 OR Unlicense OR EPL-1.0 OR EPL-2.0)',
|
2023-09-01 16:42:33 +02:00
|
|
|
}) : new AddAssetPlugin('licenses.txt', `Licenses are disabled during development`),
|
2023-06-18 10:38:47 +02:00
|
|
|
],
|
2020-01-14 19:02:08 +01:00
|
|
|
performance: {
|
2020-03-17 23:57:17 +01:00
|
|
|
hints: false,
|
2020-05-14 18:06:01 +02:00
|
|
|
maxEntrypointSize: Infinity,
|
|
|
|
maxAssetSize: Infinity,
|
2020-01-14 19:02:08 +01:00
|
|
|
},
|
2020-01-22 07:35:29 +01:00
|
|
|
resolve: {
|
|
|
|
symlinks: false,
|
2020-01-25 09:41:34 +01:00
|
|
|
},
|
2020-02-23 09:47:42 +01:00
|
|
|
watchOptions: {
|
|
|
|
ignored: [
|
|
|
|
'node_modules/**',
|
|
|
|
],
|
|
|
|
},
|
2020-05-14 18:06:01 +02:00
|
|
|
stats: {
|
2020-12-27 15:24:27 +01:00
|
|
|
assetsSort: 'name',
|
|
|
|
assetsSpace: Infinity,
|
|
|
|
cached: false,
|
|
|
|
cachedModules: false,
|
2020-05-14 18:06:01 +02:00
|
|
|
children: false,
|
2020-12-27 15:24:27 +01:00
|
|
|
chunkModules: false,
|
|
|
|
chunkOrigins: false,
|
|
|
|
chunksSort: 'name',
|
|
|
|
colors: true,
|
|
|
|
entrypoints: false,
|
2020-08-02 16:06:06 +02:00
|
|
|
excludeAssets: [
|
2020-12-27 15:24:27 +01:00
|
|
|
/^js\/monaco-language-.+\.js$/,
|
2023-09-01 16:42:33 +02:00
|
|
|
!isProduction && /^licenses.txt$/,
|
2022-10-10 14:02:20 +02:00
|
|
|
].filter(Boolean),
|
2020-12-27 15:24:27 +01:00
|
|
|
groupAssetsByChunk: false,
|
|
|
|
groupAssetsByEmitStatus: false,
|
|
|
|
groupAssetsByInfo: false,
|
|
|
|
groupModulesByAttributes: false,
|
|
|
|
modules: false,
|
|
|
|
reasons: false,
|
|
|
|
runtimeModules: false,
|
2020-05-14 18:06:01 +02:00
|
|
|
},
|
2019-11-13 15:52:13 +01:00
|
|
|
};
|